QPixmap¶
QPixmap is a widget used to deal with images.
The following file types are supported. Some image formats can only be ‘read’.
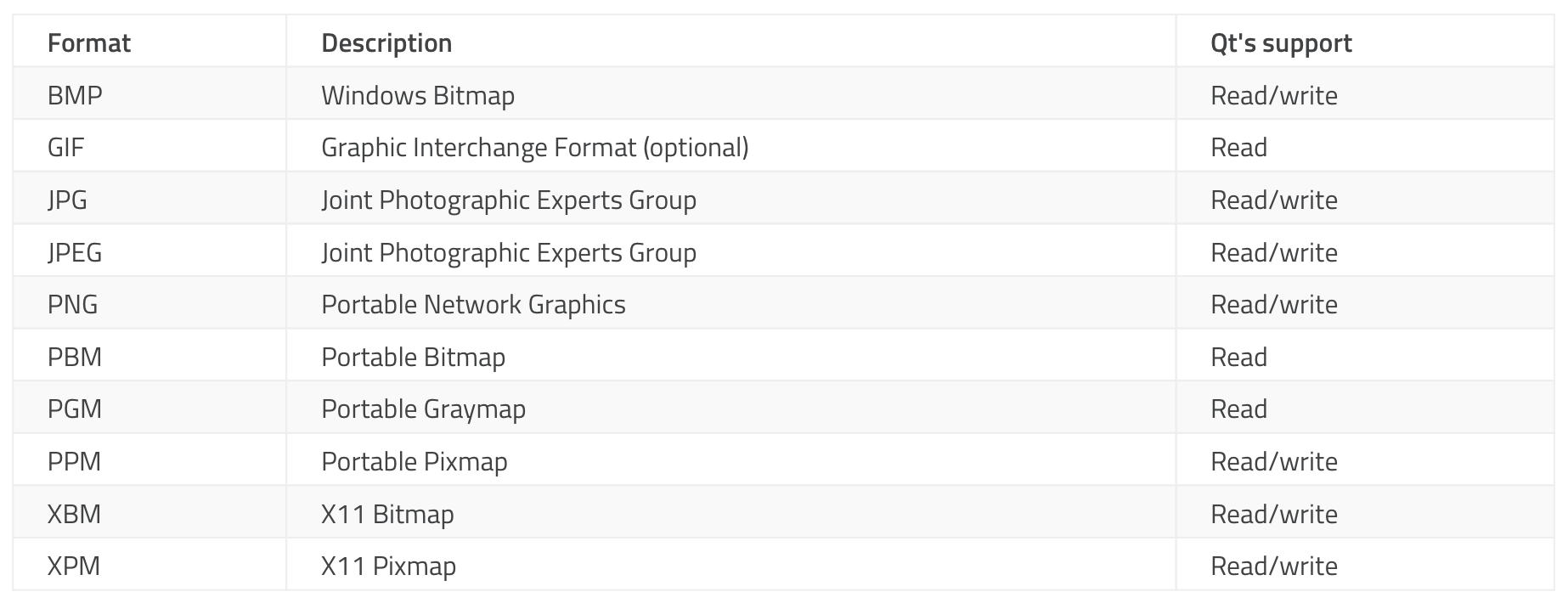
Let’s use QPixmap to display one image on the window.
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QVBoxLayout
from PyQt5.QtGui import QPixmap
from PyQt5.QtCore import Qt
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
pixmap = QPixmap('landscape.jpg')
lbl_img = QLabel()
lbl_img.setPixmap(pixmap)
lbl_size = QLabel('Width: '+str(pixmap.width())+', Height: '+str(pixmap.height()))
lbl_size.setAlignment(Qt.AlignCenter)
vbox = QVBoxLayout()
vbox.addWidget(lbl_img)
vbox.addWidget(lbl_size)
self.setLayout(vbox)
self.setWindowTitle('QPixmap')
self.move(300, 300)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
One image appears on the widget window.
Description¶
pixmap = QPixmap('landscape.jpg')
Enter a file name and create a QPixmap object.
lbl_img = QLabel()
lbl_img.setPixmap(pixmap)
Create a label and set the pixmap as the image to be displayed on the label using setPixmap.
lbl_size = QLabel('Width: '+str(pixmap.width())+', Height: '+str(pixmap.height()))
lbl_size.setAlignment(Qt.AlignCenter)
width() and height() return the image’s width and height
Create a label (lbl_size) that displays width and height, and align it to the cetner using the setAlignment method.
vbox = QVBoxLayout()
vbox.addWidget(lbl)
self.setLayout(vbox)
Create one horizontal box layout and place labels.
Use setLayout() to specify the horizontal box as the layout of the window.
Prev/Next
Prev : QTabWidget (Advanced)
Next : QCalendarWidget