Contents
- Python - 프로그래밍 시작하기
- Python 기초 (Basics)
- Python 변수 (Variables)
- Python 연산자 (Operators)
- Python 리스트 (List)
- Python 튜플 (Tuple)
- Python 문자열 (Strings)
- Python 집합 (Sets)
- Python 딕셔너리 (Dictionary)
- Python 흐름 제어 (Flow control)
- Python 함수 (Function)
- Python 클래스 (Class)
- Python 내장 함수 (Built-in function)
- Python 키워드 (Keyword)
- Keyword - and
- Keyword - as
- Keyword - assert
- Keyword - break
- Keyword - class
- Keyword - continue
- Keyword - def
- Keyword - del
- Keyword - elif
- Keyword - else
- Keyword - except
- Keyword - False
- Keyword - for
- Keyword - from
- Keyword - global
- Keyword - if
- Keyword - import
- Keyword - in
- Keyword - is
- Keyword - lambda
- Keyword - None
- Keyword - not
- Keyword - or
- Keyword - pass
- Keyword - return
- Keyword - True
- Keyword - try
- Keyword - while
- Python 파일 다루기
- Python datetime 모듈
- Python time 모듈
- Python collections.deque
- Python collections.namedtuple
- Python의 선 (Zen of Python)
Tutorials
- Python Tutorial
- NumPy Tutorial
- Matplotlib Tutorial
- PyQt5 Tutorial
- BeautifulSoup Tutorial
- xlrd/xlwt Tutorial
- Pillow Tutorial
- Googletrans Tutorial
- PyWin32 Tutorial
- PyAutoGUI Tutorial
- Pyperclip Tutorial
- TensorFlow Tutorial
- Tips and Examples
Python 파일 다루기¶
파이썬을 이용해서 파일을 열고 닫거나, 텍스트를 수정하는 방법에 대해 알아봅니다.
순서는 아래와 같습니다.
우선 아래와 같은 html 텍스트 파일 (example.html)이 경로 내에 있다고 가정합니다.
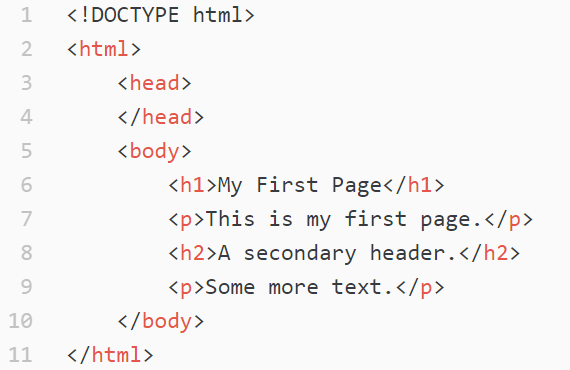
html 텍스트 파일.¶
파일 열기 (open), 닫기 (close)¶
파이썬을 이용해서 파일을 열기 위해서 파이썬 내장함수 open()
을 사용합니다.
파일을 연 후에 다시 닫아줘야 합니다. 열었던 파일을 닫을 때는 close()
를 사용합니다.
f = open('example.html')
f.close()
파일을 열 때 모드 (mode)를 설정할 수 있습니다.
f = open('example.html', 'r') # 읽기 모드 (Read) - 파일이 없으면 에러 발생. (Default)
f = open('example.html', 'a') # 추가 모드 (Append) - 파일이 없으면 생성.
f = open('example.html', 'w') # 쓰기 모드 (Write) - 파일이 없으면 생성.
f = open('example.html', 'x') # 생성 모드 (Create) - 파일이 있으면 에러 발생.
추가 모드로 파일을 열면, 기존의 내용에 새로운 텍스트를 이어서 쓸 수 있습니다.
파일 읽기 (read)¶
파일의 내용을 읽어오기 위해서 read()
, readline()
, readlines()
과 같은 다양한 함수를 사용할 수 있습니다.
read()¶
f = open('example.html')
r = f.read()
print(r)
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<h1>My First Page</h1>
<p>This is my first page.</p>
<h2>A secondary header.</h2>
<p>Some more text.</p>
</body>
</html>
f.read()
를 출력하면 전체 텍스트 파일의 내용이 출력됩니다.
f = open('example.html')
r = f.read(20)
print(r)
<!DOCTYPE html>
<htm
read(n)
과 같이 숫자를 입력해주면, 앞에서 n개의 문자를 읽습니다.
readline()¶
f = open('example.html')
r = f.readline()
print(r)
<!DOCTYPE html>
readline()
은 텍스트 파일의 한 줄을 읽습니다.
f = open('example.html')
print(f.readline())
print(f.readline())
print(f.readline())
<!DOCTYPE html>
<html>
<head>
readline()
을 사용할 때마다 한 줄씩 읽어옵니다.
f = open('example.html')
print(f.readline(10))
<!DOCTYPE
readline(n)
과 같이 숫자를 입력해주면, 그 줄의 앞에서 n개의 문자를 읽어옵니다.
readlines()¶
f = open('example.html')
print(f.readlines())
print(type(f.readlines()))
['<!DOCTYPE html>\n', '<html>\n', ' <head>\n', ' </head>\n', ' <body>\n', ' <h1>My First Page</h1>\n', ' <p>This is my first page.</p>\n', ' <h2>A secondary header.</h2>\n', ' <p>Some more text.</p>\n', ' </body>\n', '</html>\n']
<class 'list'>
readlines()
는 텍스트 파일을 리스트의 형태로 가져옵니다.
f = open('example.html')
lines = f.readlines()
for line in lines:
print(line) # '\n' 포함해서 출력
# print(line[:-1]) # '\n' 제외하고 출력
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<h1>My First Page</h1>
<p>This is my first page.</p>
<h2>A secondary header.</h2>
<p>Some more text.</p>
</body>
</html>
readlines()
와 for loop를 이용해서 텍스트 파일의 내용을 한 줄씩 처리할 수 있습니다.
한 줄의 텍스트에는 ‘<!DOCTYPE html>\n’와 같이 줄바꿈 이스케이프 문자 \n가 포함되어 있습니다.
print(line[:-1])
과 같이 사용하면, 줄 사이에 공백 없이 출력할 수 있습니다.
파일 쓰기 (write)¶
파일에 새로운 내용을 작성하기 위해서 write()
를 사용합니다.
write()¶
f = open('example_new.html', 'w') # 쓰기 모드로 파일 열기
f.write('<!DOCTYPE html>\n') # 텍스트 쓰기
f.write('\t<html>\n') # 텍스트 쓰기
f.close() # 파일 닫기
f = open('example_new.html', 'r')
print(f.read())
<!DOCTYPE html>
<html>
with 문 사용하기¶
with open('example_new.html', 'w') as f:
f.write('<!DOCTYPE html>\n')
f.write('\t<html>\n')
파일을 열고 닫을 때 위의 예제와 같이 with 키워드를 사용하면 더 편리합니다.
open()
과 close()
를 사용하는 대신 with 문이 종료되면서 파일이 자동으로 닫힙니다.
파일 삭제하기 (delete)¶
생성한 파일을 삭제하기 위해서 os 모듈을 사용합니다.
import os
os.remove('example_new.html')
os.remove()
를 실행하면 해당 파일이 삭제됩니다.
하지만 파일이 없을 경우 아래와 같은 에러가 발생합니다.
Traceback (most recent call last):
File "C:/python/test.py", line 3, in <module>
os.remove('example_new.html')
FileNotFoundError: [WinError 2] 지정된 파일을 찾을 수 없습니다: 'example_new.html'
이러한 에러를 방지하기 위해서, 아래와 같이 코드를 작성합니다.
import os
if os.path.exists("example_new.html"):
os.remove("example_new.html")
else:
print("The file does not exist")
The file does not exist