Basic usage - xlrd¶
Learn how to read Excel data using xlrd.
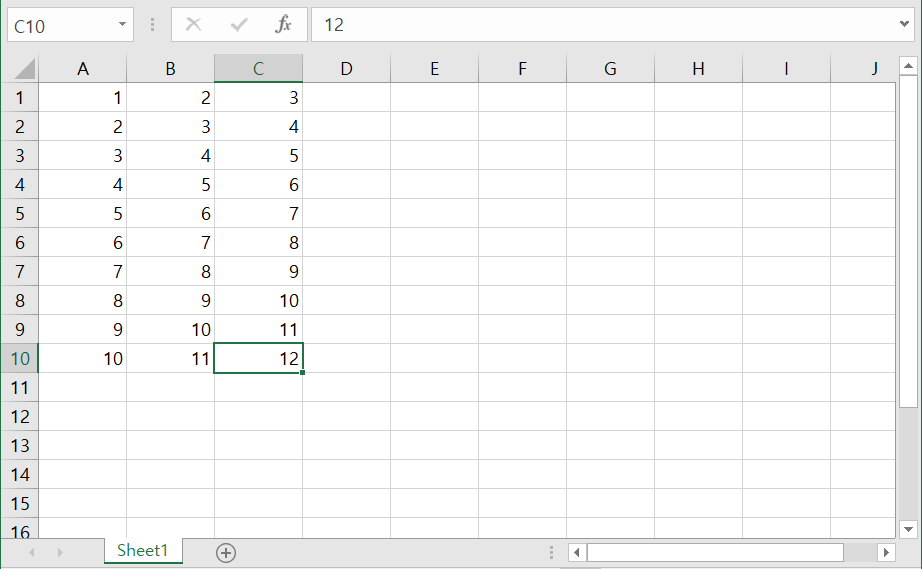
Prepare the Excel file with the data as shown in the figure above.
Example1 - Number of sheets¶
import xlrd
wb = xlrd.open_workbook('xlrd_xlwt_ex00.xls')
nsheets = wb.nsheets
print('Number of sheets:', nsheets)
Number of sheets: 1
xlrd.open_workbook()
opens the Excel file (workbook).
nsheets
are the number of sheets in the Excel file. If you print it out, you’ll see that there’s one sheet
Example2 - Number of rows and columns¶
import xlrd
wb = xlrd.open_workbook('xlrd_xlwt_ex00.xls')
sheets = wb.sheets()
nrows, ncols = sheets[0].nrows, sheets[0].ncols
print('Number of rows:', nrows)
print('Number of columns:', ncols)
Number of rows: 10
Number of columns: 3
sheets()
in the workbook(wb) returns information about the entire sheet in the form of the list.
On the first sheet (sheets[0]
), nrows and ncols are the number of rows and columns, respectively
There are 10 rows and 3 columns.
Example3 - Values in rows and columns and cells¶
import xlrd
wb = xlrd.open_workbook('xlrd_xlwt_ex00.xls')
sheets = wb.sheets()
first_row = sheets[0].row_values(0)
third_column = sheets[0].col_values(2)
cell_value_3_3 = sheets[0].cell_value(2, 2)
print(first_row)
print(third_column)
print(cell_value_3_3)
[1.0, 2.0, 3.0]
[3.0, 4.0, 5.0, 6.0, 7.0, 8.0, 9.0, 10.0, 11.0, 12.0]
5.0
row_values()
imports the values from a specific row of sheets in the form of a list.
Similarly, col_values()
obtains the values from a specific column in the sheet.
cell_value()
gets the value of the cell. Enter the indexes for rows and columns in order.
Prev/Next
Next : Basic usage - xlwt