Basic usage¶
Open Image - open()¶
from PIL import Image
im = Image.open('./img/rush_hour.png')
print(im.filename)
print(im.size)
print(im.width, im.height)
print(im.format)
Use open()
to open the image.
filename is the name of the file, size is the tuple (withd, height) and width is the horizontal size, height is the vertical size, and format is the extension of the image.
The results are as follows.
./img/rush_hour.png
(316, 563)
316 563
PNG
Crop and save image - crop(), save()¶
from PIL import Image
im = Image.open('./img/rush_hour.png')
im2 = im.crop((0, 200, 316, 510))
im2.save('./img/rush_hour_crop.png')
Use crop()
to cut certain areas of the image.
If you enter the (top left x, top left y, bottom right x, bottom right y) coordinates in the form of a tuple, the image is cut into a rectangle.
Use save()
to save the cropped image to a file. Enter the path and name of the file to be saved (‘./img/rush_hour_crop.png’).
The following image file is saved:
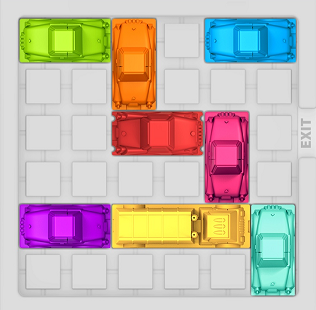
rush_hour_crop.png¶
Rotate image - rotate()¶
from PIL import Image
im = Image.open('./img/rush_hour.png')
im2 = im.crop((0, 200, 316, 510))
im2.save('./img/rush_hour_crop.png')
im3 = im2.rotate(90)
im3.save('./img/rush_hour_rotate_90.png')
im4 = im2.rotate(45)
im4.save('./img/rush_hour_rotate_45.png')
Use rotate()
to rotate the image. Let’s rotate the cropped image (im2).
For the rotate()
, enter the angle to rotate. When you rotate 90 degrees, you will see the image below.
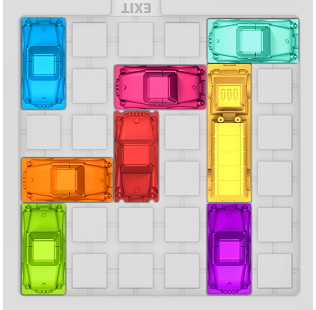
rush_hour_rotate_90.png¶
Rotating 45 degrees gives you the image below.
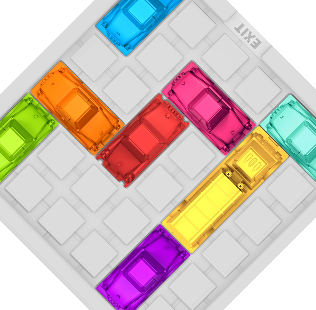
rush_hour_rotate_45.png¶