Pixel Access¶
Obtain RGB - load()¶
from PIL import Image
im = Image.open('./img/rush_hour.png')
px = im.load()
print(px[100, 50])
Use load()
to obtain the color of each pixel.
print(px[100, 50]) prints colors in x=100, y=50 of the image.
(202, 56, 70, 255)
The rgba values are printed in order.
Change color¶
from PIL import Image
im = Image.open('./rush_hour.png')
px = im.load()
for i in range(0, 316):
for j in range(200, 510):
px[i, j] = (255, 255, 255)
im.save('./rush_hour_edit.png')
You can change the color of the image pixel by changing the value of the px
.
Change pixel color from x = 0 to 315, y = 200 to 509 to white (255, 255, 255) and save as an image ‘rush_hour_edit.png’
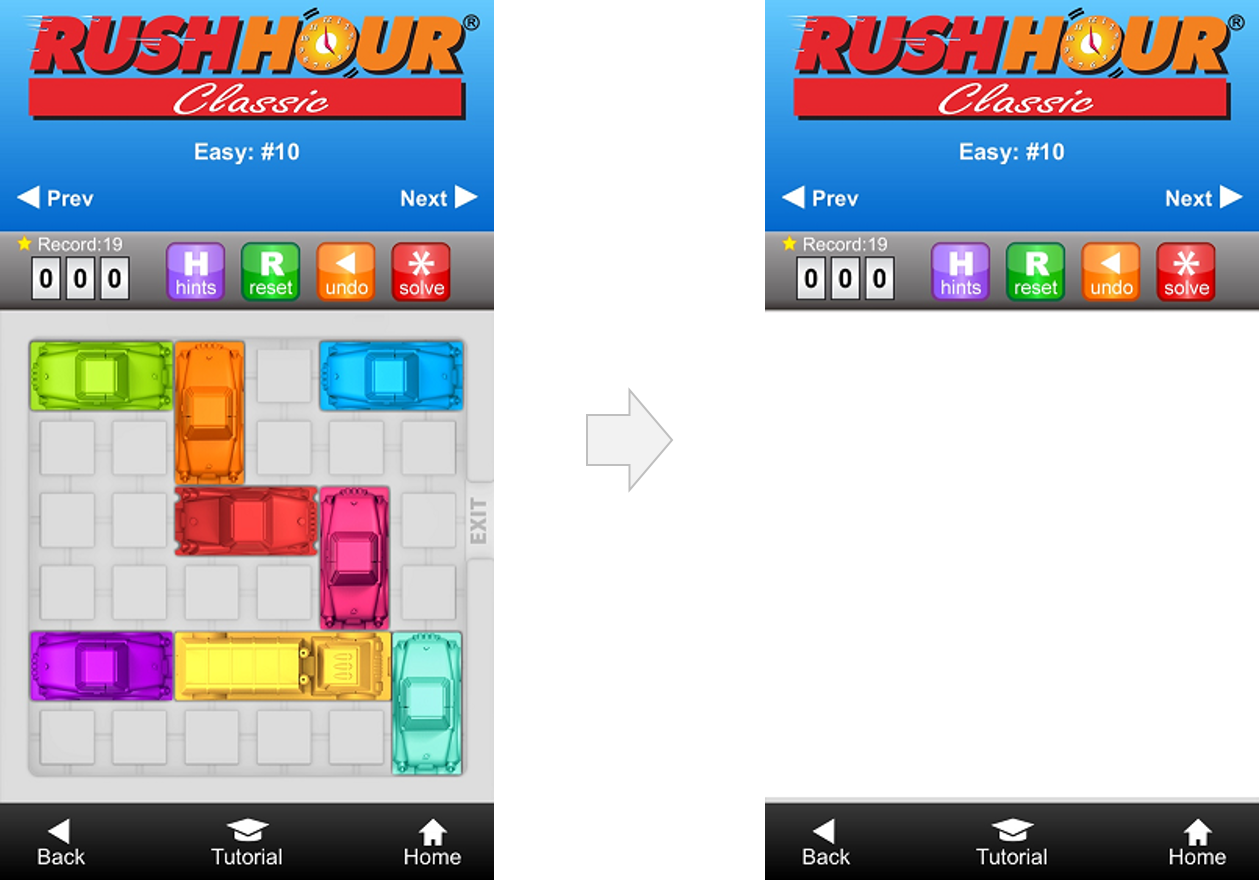
The left picture (rush_hour.png) is modified and stored as shown in the right picture (rush_hour_edit.png).
Prev
Prev : Basic usage