Contents
- Matplotlib Tutorial - 파이썬으로 데이터 시각화하기
- Matplotlib 설치하기
- Matplotlib 기본 사용
- Matplotlib 숫자 입력하기
- Matplotlib 축 레이블 설정하기
- Matplotlib 범례 표시하기
- Matplotlib 축 범위 지정하기
- Matplotlib 선 종류 지정하기
- Matplotlib 마커 지정하기
- Matplotlib 색상 지정하기
- Matplotlib 그래프 영역 채우기
- Matplotlib 축 스케일 지정하기
- Matplotlib 여러 곡선 그리기
- Matplotlib 그리드 설정하기
- Matplotlib 눈금 표시하기
- Matplotlib 타이틀 설정하기
- Matplotlib 수평선/수직선 표시하기
- Matplotlib 막대 그래프 그리기
- Matplotlib 수평 막대 그래프 그리기
- Matplotlib 산점도 그리기
- Matplotlib 3차원 산점도 그리기
- Matplotlib 히스토그램 그리기
- Matplotlib 에러바 표시하기
- Matplotlib 파이 차트 그리기
- Matplotlib 히트맵 그리기
- Matplotlib 여러 개의 그래프 그리기
- Matplotlib 컬러맵 설정하기
- Matplotlib 텍스트 삽입하기
- Matplotlib 수학적 표현 사용하기
- Matplotlib 그래프 스타일 설정하기
- Matplotlib 이미지 저장하기
- Matplotlib 객체 지향 인터페이스 1
- Matplotlib 객체 지향 인터페이스 2
- Matplotlib 축 위치 조절하기
- Matplotlib 이중 Y축 표시하기
- Matplotlib 두 종류의 그래프 그리기
- Matplotlib 박스 플롯 그리기
- Matplotlib 바이올린 플롯 그리기
- Matplotlib 다양한 도형 삽입하기
- Matplotlib 다양한 패턴 채우기
- Matplotlib 애니메이션 사용하기 1
- Matplotlib 애니메이션 사용하기 2
- Matplotlib 3차원 Surface 표현하기
- Matplotlib 트리맵 그리기 (Squarify)
- Matplotlib Inset 그래프 삽입하기
Tutorials
- Python Tutorial
- NumPy Tutorial
- Matplotlib Tutorial
- PyQt5 Tutorial
- BeautifulSoup Tutorial
- xlrd/xlwt Tutorial
- Pillow Tutorial
- Googletrans Tutorial
- PyWin32 Tutorial
- PyAutoGUI Tutorial
- Pyperclip Tutorial
- TensorFlow Tutorial
- Tips and Examples
Matplotlib 이미지 저장하기¶
matplotlib.pyplot 모듈의 savefig() 함수를 사용해서 그래프를 이미지 파일 등으로 저장하는 방법을 소개합니다.
■ Table of Contents
1) 기본 사용¶
예제¶
import numpy as np
import matplotlib.pyplot as plt
x1 = np.linspace(0.0, 5.0)
x2 = np.linspace(0.0, 2.0)
y1 = np.cos(2 * np.pi * x1) * np.exp(-x1)
y2 = np.cos(2 * np.pi * x2)
plt.subplot(2, 1, 1) # nrows=2, ncols=1, index=1
plt.plot(x1, y1, 'o-')
plt.title('1st Graph')
plt.ylabel('Damped oscillation')
plt.subplot(2, 1, 2) # nrows=2, ncols=1, index=2
plt.plot(x2, y2, '.-')
plt.title('2nd Graph')
plt.xlabel('time (s)')
plt.ylabel('Undamped')
plt.tight_layout()
# plt.show()
plt.savefig('savefig_default.png')
plt.savefig() 함수에 파일 이름을 입력해주면 아래와 같은 이미지 파일이 저장됩니다.
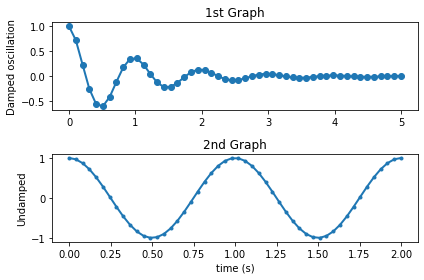
Matplotlib 이미지 저장하기 - 기본 사용¶
2) dpi 설정하기¶
3) facecolor 설정하기¶
4) edgecolor 설정하기¶
예제¶
import numpy as np
import matplotlib.pyplot as plt
plt.figure(linewidth=2)
x1 = np.linspace(0.0, 5.0)
x2 = np.linspace(0.0, 2.0)
y1 = np.cos(2 * np.pi * x1) * np.exp(-x1)
y2 = np.cos(2 * np.pi * x2)
plt.subplot(2, 1, 1) # nrows=2, ncols=1, index=1
plt.plot(x1, y1, 'o-')
plt.title('1st Graph')
plt.ylabel('Damped oscillation')
plt.subplot(2, 1, 2) # nrows=2, ncols=1, index=2
plt.plot(x2, y2, '.-')
plt.title('2nd Graph')
plt.xlabel('time (s)')
plt.ylabel('Undamped')
# plt.show()
plt.savefig('savefig_edgecolor.png', facecolor='#eeeeee', edgecolor='blue')
edgecolor는 이미지의 테두리선의 색상을 설정합니다.
테두리선의 너비가 기본적으로 0이기 때문에 먼저 linewidth=2로 설정해 준 다음 테두리 색을 지정합니다.
아래와 같은 이미지가 저장됩니다.
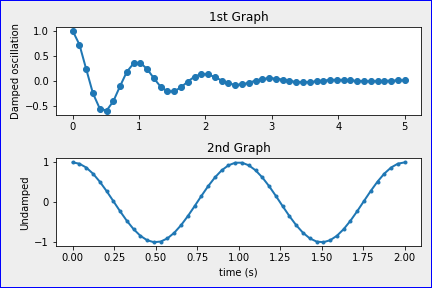
Matplotlib 이미지 저장하기 - edgecolor 설정하기¶
5) bbox_inches 설정하기¶
예제¶
plt.savefig('savefig_bbox_inches.png', facecolor='#eeeeee')
plt.savefig('savefig_bbox_inches2.png', facecolor='#eeeeee', bbox_inches='tight')
bbox_inches는 그래프로 저장할 영역을 설정합니다.
디폴트로 None이지만, ‘tight’로 지정하면 여백을 최소화하고 그래프 영역만 이미지로 저장합니다.
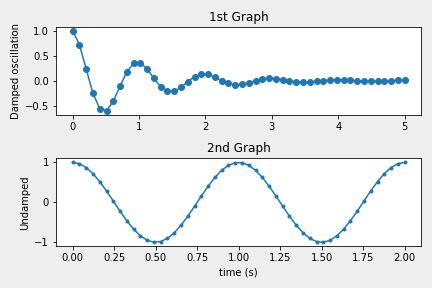
Matplotlib 이미지 저장하기 - bbox_inches 설정하기 (디폴트)¶
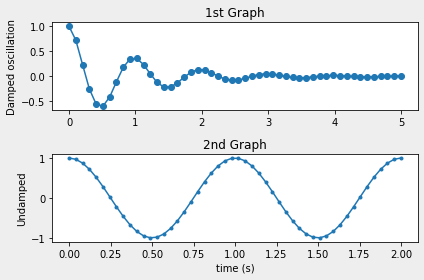
Matplotlib 이미지 저장하기 - bbox_inches 설정하기 (tight)¶
6) pad_inches 설정하기¶
예제¶
plt.savefig('savefig_pad_inches.png', facecolor='#eeeeee',
bbox_inches='tight', pad_inches=0.3)
plt.savefig('savefig_pad_inches2.png', facecolor='#eeeeee',
bbox_inches='tight', pad_inches=0.5)
bbox_inches=’tight’로 지정하면 pad_inches를 함께 사용해서 여백 (Padding)을 지정할 수 있습니다.
pad_inches의 디폴트 값은 0.1이며, 0.3과 0.5로 지정했을 때의 결과는 아래와 같습니다.
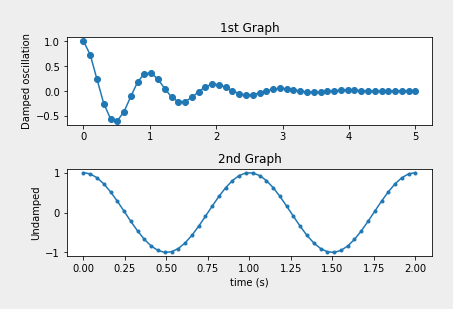
Matplotlib 이미지 저장하기 - pad_inches 설정하기 (0.3)¶
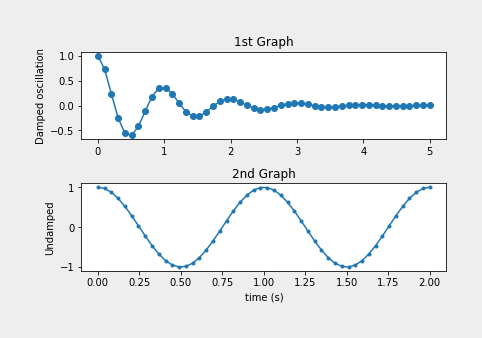
Matplotlib 이미지 저장하기 - pad_inches 설정하기 (0.5)¶
이전글/다음글
이전글 : Matplotlib 그래프 스타일 설정하기
다음글 : Matplotlib 객체 지향 인터페이스 1