Contents
- Matplotlib Tutorial - 파이썬으로 데이터 시각화하기
- Matplotlib 설치하기
- Matplotlib 기본 사용
- Matplotlib 숫자 입력하기
- Matplotlib 축 레이블 설정하기
- Matplotlib 범례 표시하기
- Matplotlib 축 범위 지정하기
- Matplotlib 선 종류 지정하기
- Matplotlib 마커 지정하기
- Matplotlib 색상 지정하기
- Matplotlib 그래프 영역 채우기
- Matplotlib 축 스케일 지정하기
- Matplotlib 여러 곡선 그리기
- Matplotlib 그리드 설정하기
- Matplotlib 눈금 표시하기
- Matplotlib 타이틀 설정하기
- Matplotlib 수평선/수직선 표시하기
- Matplotlib 막대 그래프 그리기
- Matplotlib 수평 막대 그래프 그리기
- Matplotlib 산점도 그리기
- Matplotlib 3차원 산점도 그리기
- Matplotlib 히스토그램 그리기
- Matplotlib 에러바 표시하기
- Matplotlib 파이 차트 그리기
- Matplotlib 히트맵 그리기
- Matplotlib 여러 개의 그래프 그리기
- Matplotlib 컬러맵 설정하기
- Matplotlib 텍스트 삽입하기
- Matplotlib 수학적 표현 사용하기
- Matplotlib 그래프 스타일 설정하기
- Matplotlib 이미지 저장하기
- Matplotlib 객체 지향 인터페이스 1
- Matplotlib 객체 지향 인터페이스 2
- Matplotlib 축 위치 조절하기
- Matplotlib 이중 Y축 표시하기
- Matplotlib 두 종류의 그래프 그리기
- Matplotlib 박스 플롯 그리기
- Matplotlib 바이올린 플롯 그리기
- Matplotlib 다양한 도형 삽입하기
- Matplotlib 다양한 패턴 채우기
- Matplotlib 애니메이션 사용하기 1
- Matplotlib 애니메이션 사용하기 2
- Matplotlib 3차원 Surface 표현하기
- Matplotlib 트리맵 그리기 (Squarify)
- Matplotlib Inset 그래프 삽입하기
Tutorials
- Python Tutorial
- NumPy Tutorial
- Matplotlib Tutorial
- PyQt5 Tutorial
- BeautifulSoup Tutorial
- xlrd/xlwt Tutorial
- Pillow Tutorial
- Googletrans Tutorial
- PyWin32 Tutorial
- PyAutoGUI Tutorial
- Pyperclip Tutorial
- TensorFlow Tutorial
- Tips and Examples
Matplotlib 여러 개의 그래프 그리기¶
matplotlib.pyplot 모듈의 subplot() 함수를 이용하면 여러 개의 그래프를 하나의 그림으로 나타낼 수 있습니다.
기본 사용¶
예제1¶
import numpy as np
import matplotlib.pyplot as plt
x1 = np.linspace(0.0, 5.0)
x2 = np.linspace(0.0, 2.0)
y1 = np.cos(2 * np.pi * x1) * np.exp(-x1)
y2 = np.cos(2 * np.pi * x2)
plt.subplot(2, 1, 1) # nrows=2, ncols=1, index=1
plt.plot(x1, y1, 'o-')
plt.title('1st Graph')
plt.ylabel('Damped oscillation')
plt.subplot(2, 1, 2) # nrows=2, ncols=1, index=2
plt.plot(x2, y2, '.-')
plt.title('2nd Graph')
plt.xlabel('time (s)')
plt.ylabel('Undamped')
plt.tight_layout()
plt.show()
우선 NumPy 함수를 사용해서 두 개의 cosine 함수 y1, y2를 만듭니다.
subplot(nrows, ncols, index)의 순서대로 nrows=2, ncols=1을 입력하고,
y1 함수는 index=1, y2 함수는 index=2를 입력해서 각각 위, 아래에 위치하도록 합니다.
결과는 아래와 같습니다.
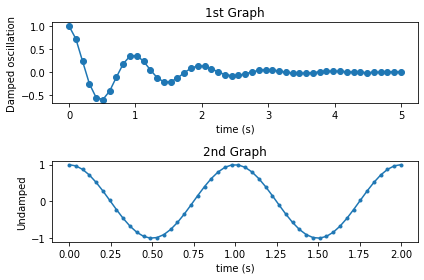
Matplotlib 여러 개의 그래프 그리기 - 기본 사용¶
예제2¶
import numpy as np
import matplotlib.pyplot as plt
x1 = np.linspace(0.0, 5.0)
x2 = np.linspace(0.0, 2.0)
y1 = np.cos(2 * np.pi * x1) * np.exp(-x1)
y2 = np.cos(2 * np.pi * x2)
plt.subplot(1, 2, 1) # nrows=1, ncols=2, index=1
plt.plot(x1, y1, 'o-')
plt.title('1st Graph')
plt.xlabel('time (s)')
plt.ylabel('Damped oscillation')
plt.subplot(1, 2, 2) # nrows=1, ncols=2, index=2
plt.plot(x2, y2, '.-')
plt.title('2nd Graph')
plt.xlabel('time (s)')
plt.ylabel('Undamped')
plt.tight_layout()
plt.show()
두 그래프를 수평 방향으로 배치하기 위해서 subplot(nrows, ncols, index)의 순서대로 nrows=1, ncols=2을 입력하고,
y1 함수는 index=1, y2 함수는 index=2를 입력해서 각각 좌우로 위치하도록 합니다.
결과는 아래와 같습니다.
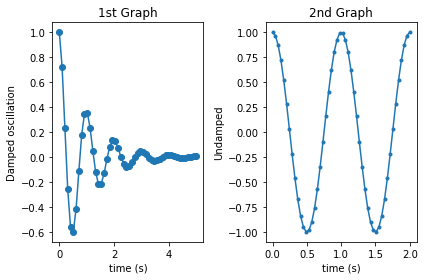
Matplotlib 여러 개의 그래프 그리기 - 기본 사용2¶