Closing window¶
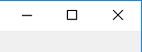
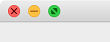
The easiest way to close a window is to click the ‘X’ button on the right(Windows) or left(macOS) of the title bar. Now we’ll learn how to close a window through programming.
We’ll also briefly learn about signal and slot.
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton
from PyQt5.QtCore import QCoreApplication
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
btn = QPushButton('Quit', self)
btn.move(50, 50)
btn.resize(btn.sizeHint())
btn.clicked.connect(QCoreApplication.instance().quit)
self.setWindowTitle('Quit Button')
self.setGeometry(300, 300, 300, 200)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
We made the ‘Quit’ button. Now if you click this button, you can quit the application.
Description¶
from PyQt5.QtCore import QCoreApplication
Import QCoreApplication class from QtCore module.
btn = QPushButton('Quit', self)
Create a push button. This button(btn) is the instance for QPushButton class.
Enter the text you wish to be shown on the button at the first parameter of (QPushButton()), and enter the parent widget in which the button will be located at the second parameter.
For further details about push button widget, refer to QPushButton page.
btn.clicked.connect(QCoreApplication.instance().quit)
PIn PyQt5, event processing works with signal and slot mechanism. If you click (btn), the ‘clicked’ signal will be created.
instance() method restores current instance.
‘clicked’ signal connects to quit()method which quits the application.
This is how the sender and receiver communicate. In this example, the sender is the push button(btn) and the receiver is the application object(app).