Opening a window¶
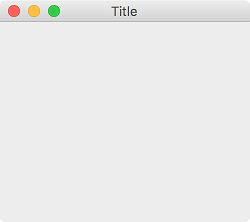
Let’s pop up a small window like the above.
You can maximize, minimize, or exit the window with the basic buttons given. You can also move the window around or resize it with your mouse.
Although these functions actually require a large number of codes, they are already coded because these functions are widely used in most applications.
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('My First Application')
self.move(300, 300)
self.resize(400, 200)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
These lines of codes display a small window on the screen.
Description¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget
Import the necessary parts. The widgets(classes) that compose the basic UI elements are included in the PyQt5.QtWidgets module.
You can check out further descriptions about classes included in QtWidgets at QtWidgets official document .
self.setWindowTitle('My First Application')
self.move(300, 300)
self.resize(400, 200)
self.show()
Here, self refers to MyApp object.
setWindowTitle() method sets the name of the window that appears on the title bar.
move() method moves the widget to x=300px, y=300px.
resize() method resizes the widget to width 400px, height 200px.
show() method displays the widget on the screen.
if __name__ == '__main__':
__name__ is the internal variable in which the current module’s name is saved.
If you import a code named ‘moduleA.py’ and run the example code, __name__ becomes ‘moduleA’.
If you just run the code, __name__ becomes ‘__main__’. Hence through this single line of code you can see whether the program is run manually or run through a module.
app = QApplication(sys.argv)
All PyQt5 applications need to create an application object. You can find further description about QApplication at QApplication official document .