Grid layout¶
The most common layout class is ‘grid layout’. This layout class separates the space in the widget by row and column.
Use QGridLayout class to create grid layout. ( QGridLayout official document )
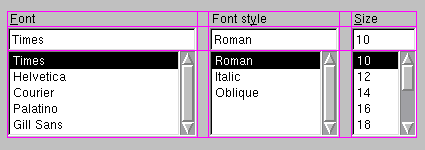
QGridLayout official document¶
For the example dialog above, it is divided into three rows and five columns, and the widget is placed at the right place.
Example¶
import sys
from PyQt5.QtWidgets import (QApplication, QWidget, QGridLayout, QLabel, QLineEdit, QTextEdit)
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
grid = QGridLayout()
self.setLayout(grid)
grid.addWidget(QLabel('Title:'), 0, 0)
grid.addWidget(QLabel('Author:'), 1, 0)
grid.addWidget(QLabel('Review:'), 2, 0)
grid.addWidget(QLineEdit(), 0, 1)
grid.addWidget(QLineEdit(), 1, 1)
grid.addWidget(QTextEdit(), 2, 1)
self.setWindowTitle('QGridLayout')
self.setGeometry(300, 300, 300, 200)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
Three labels, two line editors, and one text editor were placed in grid form.
Description¶
grid = QGridLayout()
self.setLayout(grid)
Make QGridLayout and set it as the layout for application window.
grid.addWidget(QLabel('Title:'), 0, 0)
grid.addWidget(QLabel('Author:'), 1, 0)
grid.addWidget(QLabel('Review:'), 2, 0)
Enter the widget you want to add in the first parameter in the addWidget() method, enter the row and column numbers in the second and third parameters enter, respectively.
Place the three labels vertically in the first column.
grid.addWidget(QTextEdit(), 2, 1)
Unlike the QLineEdit() widget, QTextEdit() widget is a widget that can edit many lines of text.