QSplitter¶
QSplitter class implements the splitter widget. Splitter allows you to resize child widgets’ size by dragging the boundaries.
Let’s split the widget into four smaller areas through an example.
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QHBoxLayout, QFrame, QSplitter
from PyQt5.QtCore import Qt
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
hbox = QHBoxLayout()
top = QFrame()
top.setFrameShape(QFrame.Box)
midleft = QFrame()
midleft.setFrameShape(QFrame.StyledPanel)
midright = QFrame()
midright.setFrameShape(QFrame.Panel)
bottom = QFrame()
bottom.setFrameShape(QFrame.WinPanel)
bottom.setFrameShadow(QFrame.Sunken)
splitter1 = QSplitter(Qt.Horizontal)
splitter1.addWidget(midleft)
splitter1.addWidget(midright)
splitter2 = QSplitter(Qt.Vertical)
splitter2.addWidget(top)
splitter2.addWidget(splitter1)
splitter2.addWidget(bottom)
hbox.addWidget(splitter2)
self.setLayout(hbox)
self.setGeometry(300, 300, 300, 200)
self.setWindowTitle('QSplitter')
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
The window is divided into four small areas. You can set different styles for the frames of each area.
Description¶
top = QFrame()
top.setFrameShape(QFrame.Box)
midleft = QFrame()
midleft.setFrameShape(QFrame.StyledPanel)
midright = QFrame()
midright.setFrameShape(QFrame.Panel)
bottom = QFrame()
bottom.setFrameShape(QFrame.WinPanel)
bottom.setFrameShadow(QFrame.Sunken)
Create frames for each area. You can set the shape of the frame and style of the shadow using setFrameShape and setFrameShadow.
As shown in the example, you can use NoFrame/Box/Panel/ScheduledPanel/HLine/VLine/WinPanel for setFrameShadow, and the Plain/Raised/Sunken constant for setFrameShadow.
Refer to the image below for descriptions and results of each constant’s use.
Constant that sets the shape of a frame
Constant that sets the shadow of a frame
The shape of the frame and shadow
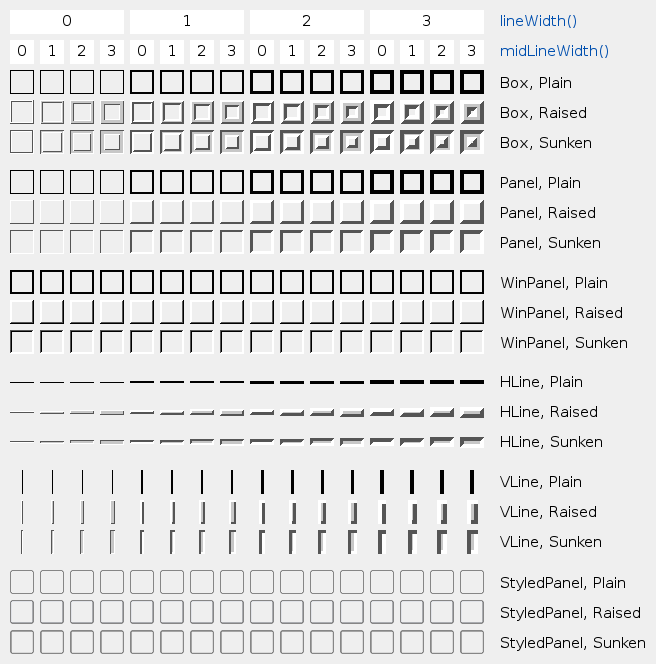
splitter1 = QSplitter(Qt.Horizontal)
splitter1.addWidget(midleft)
splitter1.addWidget(midright)
splitter2 = QSplitter(Qt.Vertical)
splitter2.addWidget(top)
splitter2.addWidget(splitter1)
splitter2.addWidget(bottom)
Use the QSplitter to split horizontally, and insert the midleft frame widget on the left and the midright frame widget on the right.
Next, break it vertically and insert three frame widgets: top, splitter1, and bottom.
hbox.addWidget(splitter2)
self.setLayout(hbox)
Place the splitter2 widget in the horizontal box layout and set it as the overall layout.