Displaying date and time¶
You can use QDate, QTime, QDateTime classes from QtCore module to display date and time on the application.
For more details, see link below.
Displaying date¶
The QDate class provides date-related features.
Print current date¶
First let’s use QDate class to print the date.
from PyQt5.QtCore import QDate
now = QDate.currentDate()
print(now.toString())
currentDate() method restores current date.
You can use toString() method to print current date as a string. The results are as follows.
수 1 2 2019
Set the date format¶
With the format parameter of toString() method, you can set the format of the date.
from PyQt5.QtCore import QDate, Qt
now = QDate.currentDate()
print(now.toString('d.M.yy'))
print(now.toString('dd.MM.yyyy'))
print(now.toString('ddd.MMMM.yyyy'))
print(now.toString(Qt.ISODate))
print(now.toString(Qt.DefaultLocaleLongDate))
‘d’ stands for day, ‘M’ for month, ‘y’ for year. The format of the date varies depending on the number of characters.
By typing Qt.ISODate, Qt.DefaultLocaleLongDate, you can print to the default settings for the ISO standard format or application. The results are as follows.
2.1.19
02.01.2019
수.1월.2019
2019-01-02
2019년 1월 2일 수요일
See the table below or refer to the official document for further details.
Displaying time¶
You can use QTime class to print current time.
Print current time¶
from PyQt5.QtCore import QTime
time = QTime.currentTime()
print(time.toString())
currentTime() method restores current time.
toString() method restores current time as a string. The results are as follows.
15:41:22
Set the time format¶
from PyQt5.QtCore import QTime, Qt
time = QTime.currentTime()
print(time.toString('h.m.s'))
print(time.toString('hh.mm.ss'))
print(time.toString('hh.mm.ss.zzz'))
print(time.toString(Qt.DefaultLocaleLongDate))
print(time.toString(Qt.DefaultLocaleShortDate))
‘h’ stands for hour, ‘m’ for minute, ‘s’ for seconds, and ‘z’ stands for 1/1000 seconds.
Just like what we did with date formatting, you can use Qt.DefaultLocaleLongDate or Qt.DefaultLocaleShortDate to set the format for time.
The results are as follows.
16.2.3
16.02.03
16.02.03.610
오후 4:02:03
오후 4:02
See the table below or refer to the official document for further details.
Displaying date and time¶
You can use QDateTime class to print current date and time together.
Print current date and time¶
from PyQt5.QtCore import QDateTime
datetime = QDateTime.currentDateTime()
print(datetime.toString())
currentDateTime() method restores current date and time.
toString() method restores date and time as as string.
Set date and time format¶
from PyQt5.QtCore import QDateTime, Qt
datetime = QDateTime.currentDateTime()
print(datetime.toString('d.M.yy hh:mm:ss'))
print(datetime.toString('dd.MM.yyyy, hh:mm:ss'))
print(datetime.toString(Qt.DefaultLocaleLongDate))
print(datetime.toString(Qt.DefaultLocaleShortDate))
Like the examples above, you can use ‘d’, ‘M’, ‘y’ for date and ‘h’, ‘m’, ‘s’ for time to set the format in which date and time is displayed.
Also, you can input Qt.DefaultLocaleLongDate or Qt.DefaultLocaleShortDate.
Displaying date on statusbar¶
Let’s print today’s date on the status bar.
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
from PyQt5.QtCore import QDate, Qt
class MyApp(QMainWindow):
def __init__(self):
super().__init__()
self.date = QDate.currentDate()
self.initUI()
def initUI(self):
self.statusBar().showMessage(self.date.toString(Qt.DefaultLocaleLongDate))
self.setWindowTitle('Date')
self.setGeometry(300, 300, 400, 200)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
Use currentDate() method to get today’s date and use showMessage() method to display the date on the status bar.
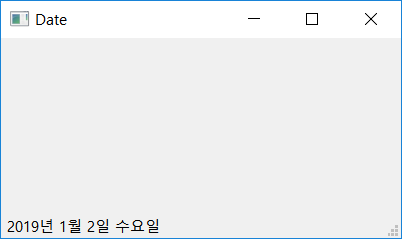
Figure 3-9. Displaying date on statusbar.