Creating a statusbar¶
The Main window is a typical application window that has menu bar, toolbar, and status bar. (QMainWindow official document 참고)
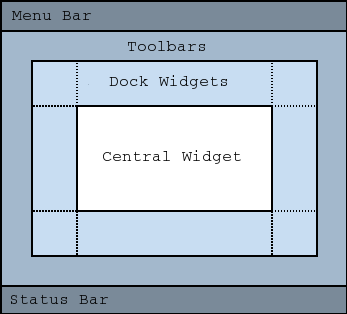
Main window has its own layout for QMenuBar, QToolBar, QDockWidget, QStatusBar. It also has a layout only for Central widget at the center in which no other widgets can be placed.
You can make main application window using QMainWindow class.

First, let’s use QStatusBar to make status bar on main window. Status bar is a widget placed at the bottom of the application to present status information of the application. ( QStatusBar official document )
To display text on the status bar, use showMessage() method. If you wish to make text disappear, use clearMessage() method or use showMessage() method to set the time for the text to be displayed.
If you want the message text that is currently being displayed on the status bar, use currentMessage() method. QStatusBar class creates messageChanged() signal whenever the message on the status bar is changed.
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
class MyApp(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.statusBar().showMessage('Ready')
self.setWindowTitle('Statusbar')
self.setGeometry(300, 300, 300, 200)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
Now a status bar is displayed below the application window.
Description¶
self.statusBar().showMessage('Ready')
The status bar is created by calling the statusBar() from QMainWindow class for the first time.
From the next call, restore the status bar object. You can use showMessage() method to set the message that will be displayed on the status bar.