Inserting an application icon¶
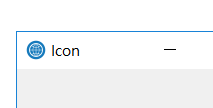
Application icon is a small image that will be shown on the left end of the title bar. Now we’ll see how to display application icon.
First, save the image file(web.png) that you wish to use as the icon in the folder.
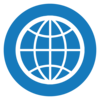
web.png¶
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtGui import QIcon
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('Icon')
self.setWindowIcon(QIcon('web.png'))
self.setGeometry(300, 300, 300, 200)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
A small icon is shown at the left end of the title bar while opening a new window.
Description¶
self.setWindowIcon(QIcon('web.png'))
setWindowIcon() method helps set up application icon. Create a QIcon object for this. Insert the image(web.png) to be displayed on QIcon().
If the image file is saved in a different folder, input the path as well.
self.setGeometry(300, 300, 300, 200)
setGeometry() method sets the window’s location and size. The first two parameters determine the window’s x,y location and the next two parameters each determine the window’s width and height.
This method is identical to combining the move() and resize() methods used in the example for opening window into a single method.