QCalendarWidget¶
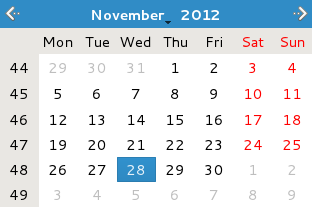
QCalendarWidget displays a calendar for the users to select a date.
The calendar is displayed monthly and is selected as the current year, month, and date when it is first run.
For further details, check QCalendarWidget official document .
Example¶
import sys
from PyQt5.QtWidgets import (QApplication, QWidget, QLabel, QVBoxLayout, QCalendarWidget)
from PyQt5.QtCore import QDate
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
cal = QCalendarWidget(self)
cal.setGridVisible(True)
cal.clicked[QDate].connect(self.showDate)
self.lbl = QLabel(self)
date = cal.selectedDate()
self.lbl.setText(date.toString())
vbox = QVBoxLayout()
vbox.addWidget(cal)
vbox.addWidget(self.lbl)
self.setLayout(vbox)
self.setWindowTitle('QCalendarWidget')
self.setGeometry(300, 300, 400, 300)
self.show()
def showDate(self, date):
self.lbl.setText(date.toString())
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
A calendar and a label that displays the date appear on the widget window.
Description¶
cal = QCalendarWidget(self)
cal.setGridVisible(True)
cal.clicked[QDate].connect(self.showDate)
Make an object(cal) of QCalenderWidget.
If you use setGridVisible(True), a grid(line) is displayed between dates.
It connects to the showDate method when you click a date.
self.lbl = QLabel(self)
date = cal.selectedDate()
self.lbl.setText(date.toString())
SelectedDate has the currently selected date information (default is the current date).
Allow current date information to be displayed on the label.
vbox = QVBoxLayout()
vbox.addWidget(cal)
vbox.addWidget(self.lbl)
Use a vertical box layout to place the calendar and label vertically.
def showDate(self, date):
self.lbl.setText(date.toString())
With the showDate method, make the label text appear as the selected date (date.toString()0 every time you click on a date.