QTabWidget (Advanced)¶
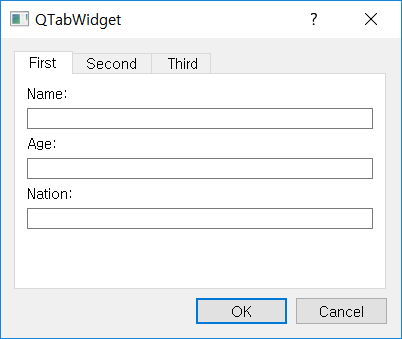
Let’s use the tab widget to configure a dialog box that allows you to receive multiple inputs in different ways.
Example¶
import sys
from PyQt5.QtWidgets import *
class MyApp(QDialog):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
tabs = QTabWidget()
tabs.addTab(FirstTab(), 'First')
tabs.addTab(SecondTab(), 'Second')
tabs.addTab(ThirdTab(), 'Third')
buttonbox = QDialogButtonBox(QDialogButtonBox.Ok | QDialogButtonBox.Cancel)
buttonbox.accepted.connect(self.accept)
buttonbox.rejected.connect(self.reject)
vbox = QVBoxLayout()
vbox.addWidget(tabs)
vbox.addWidget(buttonbox)
self.setLayout(vbox)
self.setWindowTitle('QTabWidget')
self.setGeometry(300, 300, 400, 300)
self.show()
class FirstTab(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
name = QLabel('Name:')
nameedit = QLineEdit()
age = QLabel('Age:')
ageedit = QLineEdit()
nation = QLabel('Nation:')
nationedit = QLineEdit()
vbox = QVBoxLayout()
vbox.addWidget(name)
vbox.addWidget(nameedit)
vbox.addWidget(age)
vbox.addWidget(ageedit)
vbox.addWidget(nation)
vbox.addWidget(nationedit)
vbox.addStretch()
self.setLayout(vbox)
class SecondTab(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
lan_group = QGroupBox('Select Your Language')
combo = QComboBox()
list = ['Korean', 'English', 'Chinese']
combo.addItems(list)
vbox1 = QVBoxLayout()
vbox1.addWidget(combo)
lan_group.setLayout(vbox1)
learn_group = QGroupBox('Select What You Want To Learn')
korean = QCheckBox('Korean')
english = QCheckBox('English')
chinese = QCheckBox('Chinese')
vbox2 = QVBoxLayout()
vbox2.addWidget(korean)
vbox2.addWidget(english)
vbox2.addWidget(chinese)
learn_group.setLayout(vbox2)
vbox = QVBoxLayout()
vbox.addWidget(lan_group)
vbox.addWidget(learn_group)
self.setLayout(vbox)
class ThirdTab(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
lbl = QLabel('Terms and Conditions')
text_browser = QTextBrowser()
text_browser.setText('This is the terms and conditions')
checkbox = QCheckBox('Check the terms and conditions.')
vbox = QVBoxLayout()
vbox.addWidget(lbl)
vbox.addWidget(text_browser)
vbox.addWidget(checkbox)
self.setLayout(vbox)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
A dialog with three tabs is made.
Description¶
tabs = QTabWidget()
tabs.addTab(FirstTab(), 'First')
tabs.addTab(SecondTab(), 'Second')
tabs.addTab(ThirdTab(), 'Third')
buttonbox = QDialogButtonBox(QDialogButtonBox.Ok | QDialogButtonBox.Cancel)
buttonbox.accepted.connect(self.accept)
buttonbox.rejected.connect(self.reject)
Create tabs using QTabWidget() and use addTab() to add three tabs.
Use QDialogButtonBox() to create a button box with the ‘OK’ and ‘Cancel’ buttons.
class FirstTab(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
name = QLabel('Name:')
nameedit = QLineEdit()
age = QLabel('Age:')
ageedit = QLineEdit()
nation = QLabel('Nation:')
nationedit = QLineEdit()
The FirstTab object contains three labels and three line editors.
vbox = QVBoxLayout()
vbox.addWidget(name)
vbox.addWidget(nameedit)
vbox.addWidget(age)
vbox.addWidget(ageedit)
vbox.addWidget(nation)
vbox.addWidget(nationedit)
vbox.addStretch()
self.setLayout(vbox)
Use the vertical box layout to position the six widgets vertically.
class SecondTab(QWidget):
...
lan_group = QGroupBox('Select Your Language')
combo = QComboBox()
list = ['Korean', 'English', 'Chinese']
combo.addItems(list)
Create a group box (lan_group) and place a combo box with three items: ‘Korean’, ‘English’, and ‘Chinese’.
learn_group = QGroupBox('Select What You Want To Learn')
korean = QCheckBox('Korean')
english = QCheckBox('English')
chinese = QCheckBox('Chinese')
Create three check boxes in which you can make multiple choices in the other group box (learn_group).
vbox = QVBoxLayout()
vbox.addWidget(lan_group)
vbox.addWidget(learn_group)
self.setLayout(vbox)
Place the two group boxes(lan_group, learn_group) vertically using the vertical box.
class ThirdTab(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
lbl = QLabel('Terms and Conditions')
text_browser = QTextBrowser()
text_browser.setText('This is the terms and conditions')
checkbox = QCheckBox('Check the terms and conditions.')
ThirdTab objects to be located on the third tab include a label (lbl), a text_browser, and a checkbox.
Text browsers created using QTextBrowser() are used to represent text using hypertext.
If you want to make text editable, you must use QTextEdit().
To disable hypertext and make it non-editable, you can use the setReadOnly() method in QTextEdit().
vbox = QVBoxLayout()
vbox.addWidget(lbl)
vbox.addWidget(text_browser)
vbox.addWidget(checkbox)
self.setLayout(vbox)
Use the vertical box layout to add labels, text browsers, and check boxes.