QComboBox¶
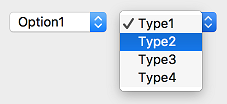
QComboBox is a widget that takes up a small amount of space, offers multiple options, and allows you to choose one of them. ( QComboBox official document )
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QComboBox
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.lbl = QLabel('Option1', self)
self.lbl.move(50, 150)
cb = QComboBox(self)
cb.addItem('Option1')
cb.addItem('Option2')
cb.addItem('Option3')
cb.addItem('Option4')
cb.move(50, 50)
cb.activated[str].connect(self.onActivated)
self.setWindowTitle('QComboBox')
self.setGeometry(300, 300, 300, 200)
self.show()
def onActivated(self, text):
self.lbl.setText(text)
self.lbl.adjustSize()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
We created a label and a combobox widget, so that the selected item in the combobox appears on the label.
Description¶
cb = QComboBox(self)
cb.addItem('Option1')
cb.addItem('Option2')
cb.addItem('Option3')
cb.addItem('Option4')
cb.move(50, 50)
We created a QComboBox widget and added four optional options using the addItem() method.
cb.activated[str].connect(self.onActivated)
When the option is selected, the onActivated() method is called.
def onActivated(self, text):
self.lbl.setText(text)
self.lbl.adjustSize()
The text of the selected item appears on the label, and with the adjustSize() method the label’s size is automatically adjusted.
Prev/Next
Prev : QRadioButton
Next : QLineEdit