QLineEdit¶
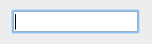
QLineEdit is a widget that lets you enter and modify a single line of string. ( QLineEdit official document )
By setting echoMode(), it can be used as a ‘write-only’ area. This feature is useful when receiving inputs such as passwords.
You can set these modes with the setEchoMode() method, and the inputs and functions are shown in the table below.
Normal mode is the most commonly used and is also the default setting (e.g. setEchoMode (QLineEdit.Normal) or setEchoMode (0)).
Constant |
Value |
Description |
---|---|---|
QLineEdit.Normal |
0 |
Display characters as they are entered. This is the default. |
QLineEdit.NoEcho |
1 |
Do not display anything. This may be appropriate for passwords where even the length of the password should be kept secret. |
QLineEdit.Password |
2 |
Display platform-dependent password mask characters instead of the characters actually entered. |
QLineEdit.PasswordEchoOnEdit |
3 |
Display characters as they are entered while editing otherwise display characters as with Password. |
You can limit the length of text entered with maxLength() method or you limit the type of text entered with setValidator() method.
You can edit text with setText() or insert() method, and import entered text with text() method. If the text entered by echoMode differs from the text displayed, you can also import the text displayed with the displayText() method.
You can select text with setSelection(), selectAll() methods, or perform actions such as cutting, copying, and pasting with cut(), copy(), paste() methods. You can also set the alignment of text with the setAlignment() method.
You can select text with setSelection(), selectAll() methods, or perform actions such as cutting, copying, and pasting with cut(), copy(), paste() methods. You can also set the alignment of text with the setAlignment() method.
Commonly used signals are shown below.
Signal |
Description |
---|---|
cursorPositionChanged() |
Generates a signal that occurs when the cursor moves. |
editingFinished() |
Generates a signal when the editing is finished (when the Return/Enter button is pressed). |
returnPressed() |
Generates a signal when the Return/Enter button is pressed. |
selectionChanged() |
Generates a signal when the selected area is changed. |
textChanged() |
Generates a signal when the text is changed. |
textEdited() |
Generates a signal when the text is edited. |
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QLineEdit
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.lbl = QLabel(self)
self.lbl.move(60, 40)
qle = QLineEdit(self)
qle.move(60, 100)
qle.textChanged[str].connect(self.onChanged)
self.setWindowTitle('QLineEdit')
self.setGeometry(300, 300, 300, 200)
self.show()
def onChanged(self, text):
self.lbl.setText(text)
self.lbl.adjustSize()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
The widget contains a label and a QLineEdit widget. The label immediately displays the text that is entered and modified in the QLineEdit widget.
Description¶
qle = QLineEdit(self)
We made the QLineEdit widget.
qle.textChanged[str].connect(self.onChanged)
When the text of qle changes, the onChanged() method is called.
def onChanged(self, text):
self.lbl.setText(text)
self.lbl.adjustSize()
Within the onChanged() method, let the entered ‘text’ be set as the text in the label widget (lbl).
Also, use the adjustSize() method to adjust the length of the label according to the length of the text.