Creating a toolbar¶
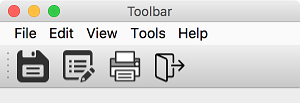
While ‘menu’ is a collection of all the commands used in the application, toolbar makes frequently used commands more convenient to use. (QToolBar official document 참고)
Save the icons for the toolbar functions(save.png, edit.png, print.png, exit.png) in the folder.
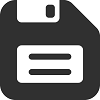
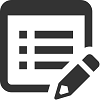
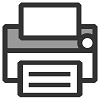
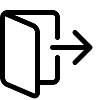
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QAction, qApp
from PyQt5.QtGui import QIcon
class MyApp(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
exitAction = QAction(QIcon('exit.png'), 'Exit', self)
exitAction.setShortcut('Ctrl+Q')
exitAction.setStatusTip('Exit application')
exitAction.triggered.connect(qApp.quit)
self.statusBar()
self.toolbar = self.addToolBar('Exit')
self.toolbar.addAction(exitAction)
self.setWindowTitle('Toolbar')
self.setGeometry(300, 300, 300, 200)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
Here we made a simple toolbar. The toolbar includes ‘exitAction’ which quits the application when selected.
Description¶
exitAction = QAction(QIcon('exit.png'), 'Exit', self)
exitAction.setShortcut('Ctrl+Q')
exitAction.setStatusTip('Exit application')
exitAction.triggered.connect(qApp.quit)
Create a QAction object like you did for menu bar. This object includes icon(exit.png) and label(‘Exit’) and can be run through shortkey(Ctrl+Q).
Show the message(‘Exit application’) on the status bar, and the signal created when you click on it is connected to the quit() method.
self.toolbar = self.addToolBar('Exit')
self.toolbar.addAction(exitAction)
Use addToolbar() to create a toolbar, and use addAction() to add exitAction to the toolbar.
Prev/Next
Prev : Creating a menubar
Next : Centering window