QSlider & QDial¶

The QSlider provides a slider in horizontal or vertical direction.
The slider is a widget that adjusts value within a limited range. ( QSlider official document )

Use the setTickInterval() method to adjust the interval of the slider’s tick, and use the setTickPosition() method to adjust the position of the tick in the slider.
The input value of the setTickInterval() method stands for value, not pixel.
The input values and functions of the setTickPosition() method are shown in the table below. (e.g.: setTickPosition(QSlider.NoTicks) or setTickPosition(0))
Constant |
Value |
Description |
---|---|---|
QSlider.NoTicks |
0 |
Do not show ticks. |
QSlider.TicksAbove |
1 |
Display the tick on top of the (horizontal) slider. |
QSlider.TicksBelow |
2 |
Display the tick on the bottom of the (horizontal) slider. |
QSlider.TicksBothSides |
3 |
Display the tick on both sides of the (horizontal) slider. |
QSlider.TicksLeft |
TicksAbove |
Display the tick on the left of the (vertical) slider. |
QSlider.TicksRight |
TicksBelow |
Display the tick on the right of the (vertical) slider. |
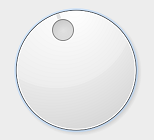
QDial is a dial widget that represents the slider in a circular form and shares the same signals, slots, and methods. ( QDial official document )
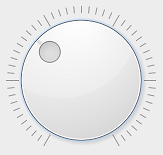
Use the setNotchesVisible() method to display notch in the Dial widget as shown above. When set to True, the notches are displayed along the round dial. By default, the notch is disabled.
The most frequently used signals in the QSlider and QDial widgets are shown below. In the example, we will use the valueChanged signal.
Value |
Description |
---|---|
valueChanged() |
Emitted when the slider’s value has changed. The tracking() determines whether this signal is emitted during user interaction. |
sliderPressed() |
Emitted when the user starts to drag the slider. |
sliderMoved() |
Emitted when the user drags the slider. |
sliderReleased() |
Emitted when the user releases the slider. |
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QSlider, QDial, QPushButton
from PyQt5.QtCore import Qt
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.slider = QSlider(Qt.Horizontal, self)
self.slider.move(30, 30)
self.slider.setRange(0, 50)
self.slider.setSingleStep(2)
self.dial = QDial(self)
self.dial.move(30, 50)
self.dial.setRange(0, 50)
btn = QPushButton('Default', self)
btn.move(35, 160)
self.slider.valueChanged.connect(self.dial.setValue)
self.dial.valueChanged.connect(self.slider.setValue)
btn.clicked.connect(self.button_clicked)
self.setWindowTitle('QSlider and QDial')
self.setGeometry(300, 300, 400, 200)
self.show()
def button_clicked(self):
self.slider.setValue(0)
self.dial.setValue(0)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
We made a slider, dial widget, and a push button. The slider and dial are linked together so that they always have the same value, and when you press the push button, both widgets’ values become 0.
Description¶
self.slider = QSlider(Qt.Horizontal, self)
We created a QSlider widget. Enter Qt.Horizontal or Qt.Enter Vertical to set the horizontal or vertical orientation.
self.slider.setRange(0, 50)
self.slider.setSingleStep(2)
setRange() method sets the range of the value from 0 to 50. The setSingleStep() method sets the minimum adjustable units.
self.dial = QDial(self)
We made a QDial widget.
self.dial.setRange(0, 50)
Just like the slider, setRange() sets the range.
self.slider.valueChanged.connect(self.dial.setValue)
self.dial.valueChanged.connect(self.slider.setValue)
The values of the two widgets are always identical by connecting the signals that occur when the values of the slider and dial change to each other’s dial and slider value adjusting method (setValue).
btn.clicked.connect(self.button_clicked)
Connect the signal that occurs when you click the ‘Default’ push button to the button_clicked method.
def button_clicked(self):
self.slider.setValue(0)
self.dial.setValue(0)
The button_clicked() method adjusts both the slider and dial values to zero. Therefore, when the ‘Default’ pushbutton is clicked, the values on the slider and dial are reset to 0.