QPushButton¶
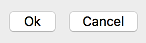
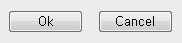
Push button, or command button, is a button that is used when a user commands the program to do a certain action, and is the most common and important widget in GUI programming. ( QPushButton official document )
You can make this with QPushButton class. The methods and signals often used with the push button are listed in the table below.
Frequently used methods
Method |
Description |
---|---|
setCheckable() |
If you set it as True, it distinguishes between the pressed and unpressed state. |
toggle() |
Changes the state. |
setIcon() |
Sets the icon of the button. |
setEnabled() |
If you set it as False, you cannot use the button. |
isChecked() |
Returns whether a button is selected or not. |
setText() |
Sets the text to be displayed on the button. |
text() |
Returns the text displayed on the button. |
Frequently used signals
Signal |
Description |
---|---|
clicked() |
Generated when a button is clicked. |
pressed() |
Generated when a button is pressed. |
released() |
Generated when a button is released. |
toggled() |
Generated when the state of the button changes. |
Now let’s create a push button using the QPushButton class.
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QVBoxLayout
from PyQt5.QtGui import QIcon, QPixmap
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
btn1 = QPushButton('&Button1', self)
btn1.setCheckable(True)
btn1.toggle()
btn2 = QPushButton(self)
btn2.setText('Button&2')
btn3 = QPushButton('Button3', self)
btn3.setEnabled(False)
vbox = QVBoxLayout()
vbox.addWidget(btn1)
vbox.addWidget(btn2)
vbox.addWidget(btn3)
self.setLayout(vbox)
self.setWindowTitle('QPushButton')
self.setGeometry(300, 300, 300, 200)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
Created three different push buttons.
Description¶
btn1 = QPushButton('&Button1', self)
btn1.setCheckable(True)
btn1.toggle()
Create a push button with PushButton class. The first parameter specifies the text to appear on the button, and the second parameter specifies the parent class to which the button belongs.
If you want to specify a shortcut on the button, simply insert the ampersand (‘&’) in front of the character as shown below. The shortcut key for this button is ‘Alt+b’.
Setting setCheckable() to True will allow you to remain the selected or unselected state.
If you call the toggle() method, the state of the button changes. Therefore, this button is selected when the program starts.
btn2 = QPushButton(self)
btn2.setText('Button&2')
The setText() method also allows you to specify the text to be displayed on the button. The shortcut key for this button will also be ‘Alt+2’.
btn3 = QPushButton('Button3', self)
btn3.setEnabled(False)
If setEnabled() is set to False, the button becomes unusable.