Displaying a tooltip¶
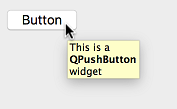
Tooltip is a short piece of text that explains a widget’s function. ( QToolTip official document )
We can make tooltip appear for all the elements in the widget.
We’ll use setToolTip() method to make tooltip in widgets.
Example¶
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QToolTip
from PyQt5.QtGui import QFont
class MyApp(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
QToolTip.setFont(QFont('SansSerif', 10))
self.setToolTip('This is a <b>QWidget</b> widget')
btn = QPushButton('Button', self)
btn.setToolTip('This is a <b>QPushButton</b> widget')
btn.move(50, 50)
btn.resize(btn.sizeHint())
self.setWindowTitle('Tooltips')
self.setGeometry(300, 300, 300, 200)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MyApp()
sys.exit(app.exec_())
In this example, we can see tooltips for two PyQt5 widgets. If you move the cursor to push button(btn) and window(MyApp) widgets, the set-up text will appear as tooltip.
Description¶
QToolTip.setFont(QFont('SansSerif', 10))
self.setToolTip('This is a <b>QWidget</b> widget')
First set the font for tooltip. Here we used 10px ‘SansSerif’.
To make tooltip, use setToolTip() method to enter the text you wish to be displayed.
btn = QPushButton('Button', self)
btn.setToolTip('This is a <b>QPushButton</b> widget')
Make a push button and add tooltip to this button as well.
btn.move(50, 50)
btn.resize(btn.sizeHint())
Adjust the button’s location and size. sizeHint() mehtod helps adjust the button to appropriate size.
Prev/Next
Prev : Closing window
Next : Creating a statusbar